PHP Form Handling
The PHP superglobals $_GET and $_POST are used to collect form-data.
• PHP - A Simple HTML Form
The example below displays a simple HTML form with two input fields and a submit button:
Example
The PHP file demo_phpfile.php:
<!DOCTYPE html> <html> <body> <form action="welcome.php" method="post"> Name: <input type="text" name="name"><br> E-mail: <input type="text" name="email"><br> <input type="submit"> </form> </body> </html>
Output
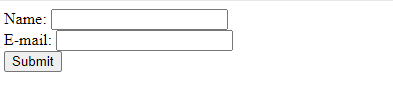
When the user fills out the form above and clicks the submit button, the form data is sent for processing to a PHP file named "welcome.php". The form data is sent with the HTTP POST method.
To display the submitted data you could simply echo all the variables. The "welcome.php" looks like this:
<html> <body> Welcome <?php echo $_POST["name"]; ?><br> Your email address is: <?php echo $_POST["email"]; ?> </body> </html>
The output could be something like this:
Welcome John
Your email address is john.doe@example.com
The same result could also be achieved using the HTTP GET method:
Example
<!DOCTYPE HTML> <html> <body> <form action="welcome_get.php" method="get"> Name: <input type="text" name="name"><br> E-mail: <input type="text" name="email"><br> <input type="submit"> </form> </body> </html>
Output
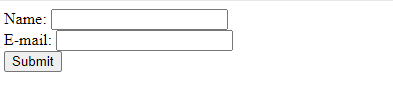
and "welcome_get.php" looks like this:
<html> <body> Welcome <?php echo $_GET["name"]; ?><br> Your email address is: <?php echo $_GET["email"]; ?> </body> </html>
• GET vs. POST
Both GET and POST create an array (e.g. array( key1 => value1, key2 => value2, key3 => value3, ...)). This array holds key/value pairs, where keys are the names of the form controls and values are the input data from the user.
Both GET and POST are treated as $_GET and $_POST. These are superglobals, which means that they are always accessible, regardless of scope - and you can access them from any function, class or file without having to do anything special.
$_GET is an array of variables passed to the current script via the URL parameters.
$_POST is an array of variables passed to the current script via the HTTP POST method.