HTML Canvas Graphics
The HTML <canvas> element is used to draw graphics on a web page using JavaScript. It provides a drawing surface where you can use JavaScript to create and manipulate graphics, animations, and interactive content. The <canvas> element is part of the HTML5 specification and is widely supported by modern web browsers.
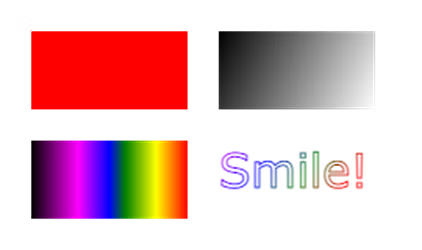
What is HTML Canvas?
The HTML <canvas> element is an HTML5 element that provides a drawing surface on a web page. It allows developers to use JavaScript to draw graphics, create animations, and build interactive content directly within the web browser. The canvas is a rectangular area on the page where you can dynamically render graphics, charts, animations, and more
Browser Support
Element | Img |
![]() |
Yes |
![]() |
Yes |
![]() |
Yes |
![]() |
Yes |
![]() |
Yes |
Canvas Examples
Certainly! Here are a few simple examples to demonstrate the usage of the HTML <canvas> element along with JavaScript for drawing graphics:
Example
<head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Canvas Example 1</title> </head> <body> <canvas id="rectangleCanvas" width="300" height="200"></canvas> <script> var canvas = document.getElementById("rectangleCanvas"); var context = canvas.getContext("2d"); // Draw a blue rectangle context.fillStyle = "blue"; context.fillRect(50, 50, 200, 100); </script> </body>You can click on above box to edit the code and run again.
Output
Add a JavaScript
After creating the rectangular canvas area, you must add a JavaScript to do the drawing.
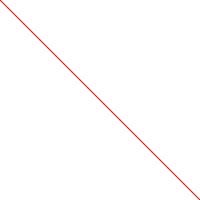
Draw a Circle
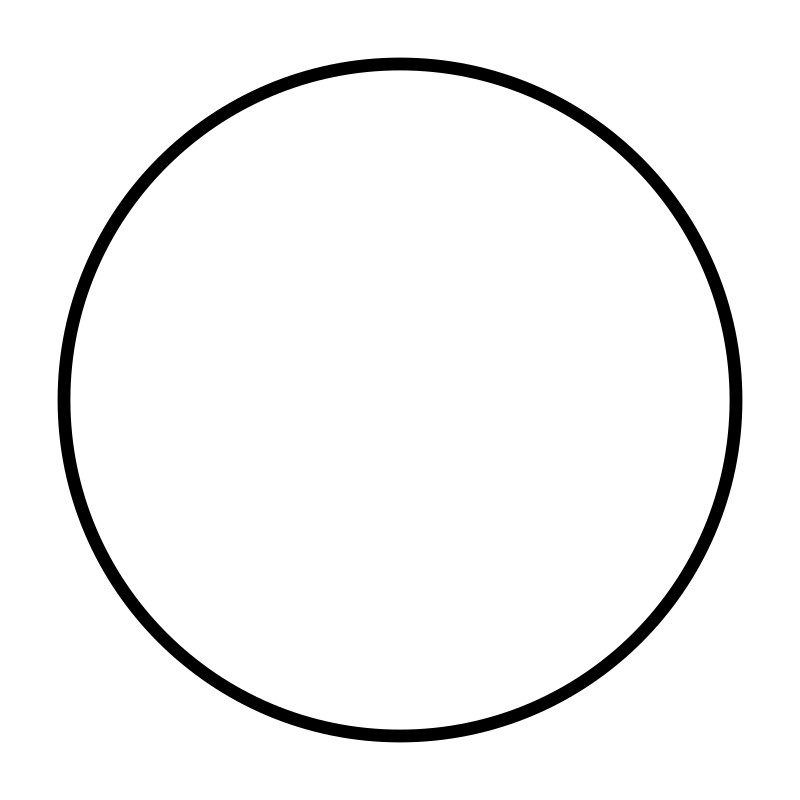
Draw a Text
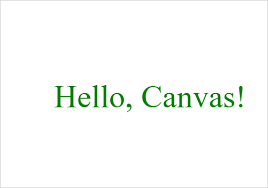
Draw Linear Gradient
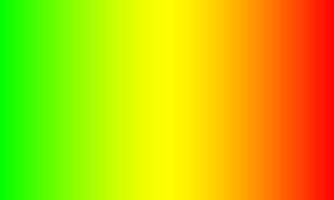
Example
<script> var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); // Create gradient var grd = ctx.createLinearGradient(0, 0, 200, 0); grd.addColorStop(0, "red"); grd.addColorStop(1, "white"); // Fill with gradient ctx.fillStyle = grd; ctx.fillRect(10, 10, 150, 80); </script>You can click on above box to edit the code and run again.
Output
Draw Circular Gradient
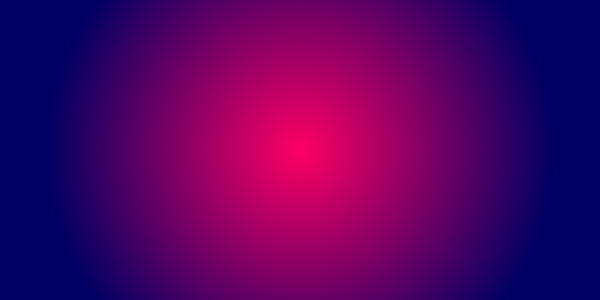
Example
<script> var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); // Create gradient var grd = ctx.createRadialGradient(75, 50, 5, 90, 60, 100); grd.addColorStop(0, "red"); grd.addColorStop(1, "white"); // Fill with gradient ctx.fillStyle = grd; ctx.fillRect(10, 10, 150, 80); </script>You can click on above box to edit the code and run again.